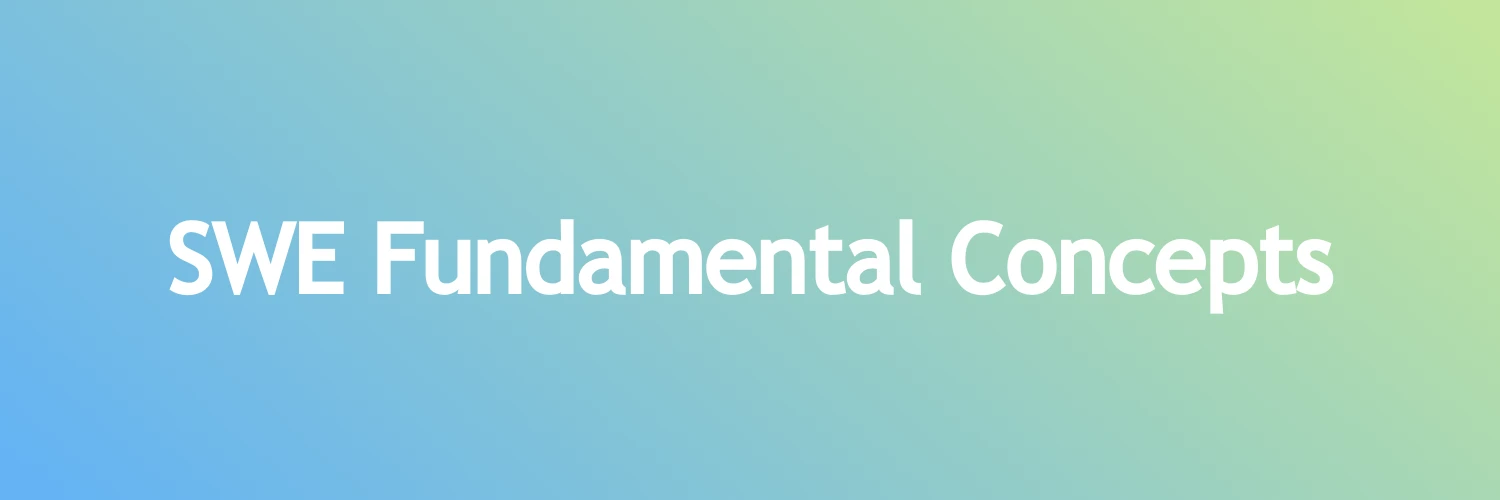
SWE Fundamental Concepts
A simple guide covering core software engineering principles, from system design and networking to databases and encryption. Bridging theoretical knowledge and practical insights, this resource serves as a fundamental reference for developers at all stages of their career.
🐸️ Introduction
In here I just wanted to share my personal note for fundamental concepts in SWE.
Interview Preparation
Answering Interview Questions
- Answer the question completely
- Share a relevant story from your past career
- Reference sample projects on GitHub that demonstrate your skills
Interview Process:
- Resume/Application
- Seek recommendations from friends or professional contacts
- Network through coffee meetings or personal introductions
- Leverage personal recommendations when possible
- Coding Challenge
- Prepare for various challenge types:
- Data structure and algorithm problems
- Multiple-choice questions
- Domain-specific expertise
- Demo app or feature development
Best Practices:- Write clean, correct code
- Break functions into small, focused units
- Use a linter
- Ensure clear problem understanding
- Add comprehensive tests and comments
- Prepare for various challenge types:
- Recruiter Phone Screen
- Approach as a friendly conversation
- Discuss company culture and role
- Be prepared to talk about salary expectations
Key Tips:- Show genuine interest in the role and company
- Read the company's blog
- Maintain a balanced, professional tone
- Ask engaging, thoughtful questions
- Technical Screening
- Expect questions on:
- Data structures and algorithms
- Method implementation
- Past project discussions
Preparation Strategies:- Deeply understand recent project challenges
- Explain problem-solving approaches
- Demonstrate language proficiency
- Communicate clearly and confidently
- Expect questions on:
💎️ Software Design Principles
Coupling and Cohesion
Cohesion
- Indicates the relationship within a module
- High cohesion is desirable
- All module items should be closely related
- Example: In a user class, all methods should relate to user functionality
Coupling
- Indicates relationships between software modules
- Low coupling is preferable
- High dependency between modules (e.g., user and post modules) suggests tight coupling
Software Requirements
Functional Requirements
- Specific end-user demands
- Basic facilities the system should offer
- Directly visible in the final product
Non-functional Requirements
- Quality constraints the system must satisfy
💎️ Processes and Threads
Process
- A program scheduled in the CPU
- Can create child processes
- Possible states: New, Ready, Running, Waiting, Terminated, Suspended
Thread
- A segment of a process
- A process can have multiple threads
- Three states: Running, Ready, Blocked
😈️ System Design Concepts
Scaling
- Vertical Scale: Adding RAM, CPU, and other resources
- Horizontal Scale: Creating multiple server instances
Key Distributed System Concepts
- Leader Election: Selecting a leader server with specific responsibilities
- Examples: Zookeeper, etcd.io
- Logging: Recording system or software events
- Publish/Subscribe: Messaging pattern where publishers send messages without direct receiver specification
- Idempotent Operations: Operations that produce the same result regardless of how many times they're performed
💎️ Networking Fundamentals
Client-Server Model
- Client: Machine requesting or sending data to a server
- Server: Machine listening to and responding to client requests
DNS
- DNS Query: Request to obtain an IP address for a domain
- DNS Server: Translates domain names to IP addresses
IP Addresses
- Unique identifier for network-connected devices
IPv4 vs IPv6
Characteristic | IPv4 | IPv6 |
---|---|---|
Address Length | 32-bit | 128-bit |
Addressing Method | Numeric | Alphanumeric |
Separator | Dot (.) | Colon (:) |
Header Fields | 12 | 8 |
Network Protocols
TCP (Transmission Control Protocol)
- Communication standard for packet transmission
- Ensures data integrity
- Establishes connection between source and destination
HTTP (Hypertext Transfer Protocol)
- Protocol built on TCP
- Request/response paradigm
- Uses port 80
- Versions:
- HTTP/1: Basic implementation
- HTTP/2: Server-push feature
- HTTP/3: Parallel transmission streams
Network Layers
- Application: HTTP, FTP, SSH, SMTP
- Transport: UDP, TCP
- Network (Internet): IP
- Link (Hardware): WiFi, Ethernet
- Physical: Physical cables
Protocol Characteristics
UDP (User Datagram Protocol)
- Lightweight (8-byte headers)
- Connectionless
- Inconsistent delivery
- Very fast
- Ideal for: Video games, video chats
- Unreliable transmission
TCP (Transmission Control Protocol)
- Connection-based
- Three-way handshake process
- Congestion control
- Reliable delivery
- Delivery acknowledgments
- Retransmission support
- In-order packet guarantee
😈️ Performance and System Characteristics
Latency and Throughput
Latency
- Definition: Time taken for data to travel from one point to another
- Various types of latency:
- Database read latency
- Network latency (request round trip)
Latency Reduction Strategies
- Implement caching
- Improve database performance
- Optimize network infrastructure
Throughput
- Measurement of work performed in a specific time period
- Typically measured in:
- GB per second
- MB per second
- Indicates system capacity (e.g., server request handling)
😈️ System Availability
Availability Concepts
- System resilience and fault tolerance
- High Availability (HA)
- Service Level Agreement (SLA)
Availability Measurement: Nines
Percentage | Nines Classification |
---|---|
90% | One nine |
99.5% | Two and a half nines |
99.9% | Three nines |
Improving System Availability
- Eliminate single points of failure
- Implement redundancy
Redundancy Types
- Passive Redundancy:
- System continues operation if a component fails
- Replication of failed parts
- Active Redundancy:
- Remaining services automatically take over failed service duties
😈️ Caching and Performance Optimization
Caching Fundamentals
- Method of storing computation results
- Quick access without repeated computation
- Primary goal: System speed optimization
😈️ Proxy Technologies
Forward Proxy
- Server intermediary between client and server
- Client requests routed through proxy
- Source IP address modified
- Example: VPN services
Reverse Proxy
- Single entity for proxy and server
- Client perceives interaction with a single server
- Hides actual server infrastructure
😈️ Load Balancing
Load Balancer Strategies
- Distribute workloads across resources
- Request distribution methods:
- Round-robin
- Weighted resource allocation
- Least-connection approach
Advanced Techniques
- Sticky-session cookies
- Ensuring consistent client-server routing
- Example: NGINX ip-hash directive
😈️ Hashing
- Transformation of arbitrary data to fixed-size value
😈️ Page Rendering Techniques
Server-Side Rendering (SSR)
- HTML rendering on client-side
- Improved SEO performance
Hydration Strategies
- Standard Hydration:
- Attaching event handlers
- Rendering on server and client
- Progressive Hydration:
- Selective component hydration
- Resumability:
- Dynamic JavaScript loading on user interaction
😈️ Database Technologies
Database Classification
Relational Databases
- Based on relational data model
- SQL (Structured Query Language) based
NoSQL Databases
- Flexible, scalable alternative to traditional databases
- No strict relational model
NoSQL Database Types
- Key-Value Databases
- Data stored as key-value pairs
- Examples: Redis, Berkeley DB, Amazon DynamoDB
- Document Databases
- Data stored as documents (JSON/XML)
- Examples: MongoDB, CouchDB, BaseX
- Column-Oriented Databases
- Data stored as strongly-typed columns
- Quick aggregation of large data volumes
- Examples: HBase, Bigtable, Cloudera
- Graph Databases
- Data stored in graph structures
- Examples: Neo4J, InfiniteGraph
Database Selection Criteria
Choose SQL When:
- Highly structured, stable data
- Transactional requirements
- Complex query needs
Choose NoSQL When:
- Large data volumes
- Dynamic schema flexibility
- Horizontal scalability requirements
Database Characteristics
ACID Properties
- Atomicity: Complete or no transaction
- Consistency: Maintain data integrity
- Isolation: Prevent transaction interference
- Durability: Committed transactions survive system failures
Specialized Database Types
- Blob Storage: Unstructured data storage
- Examples: Google Cloud Storage, S3, Minio
- Time Series DB: Event and time-relevant data
- Examples: InfluxDB, Prometheus
- Use cases: IoT, logging, monitoring
- Spatial DB: Geographic and spatial data storage
Data Management Strategies
Replication
- Multiple database node synchronization
- Benefits:
- Increased read performance
- Fault tolerance
- Asynchronous data writing
Sharding
- Data distribution across multiple servers
- Types:
- Vertical sharding: Column distribution
- Horizontal sharding: Row distribution
- Requires middleware for operation routing
😈️ Distributed Computing
MapReduce Framework
- Large dataset processing across multiple servers
- Two-phase processing:
- Map: Data splitting and key/value mapping
- Reduce: Data shuffling and reduction
😈️ Encryption Techniques
Symmetric Encryption
- Single key encryption
- Examples: AES, Triple DES
- Advanced Encryption Standard (AES)
Asymmetric Encryption
- Two-key system (public/private)
- Public key: Encryption
- Private key: Decryption
- Digital signature generation via message hash encryption
Tags:CS