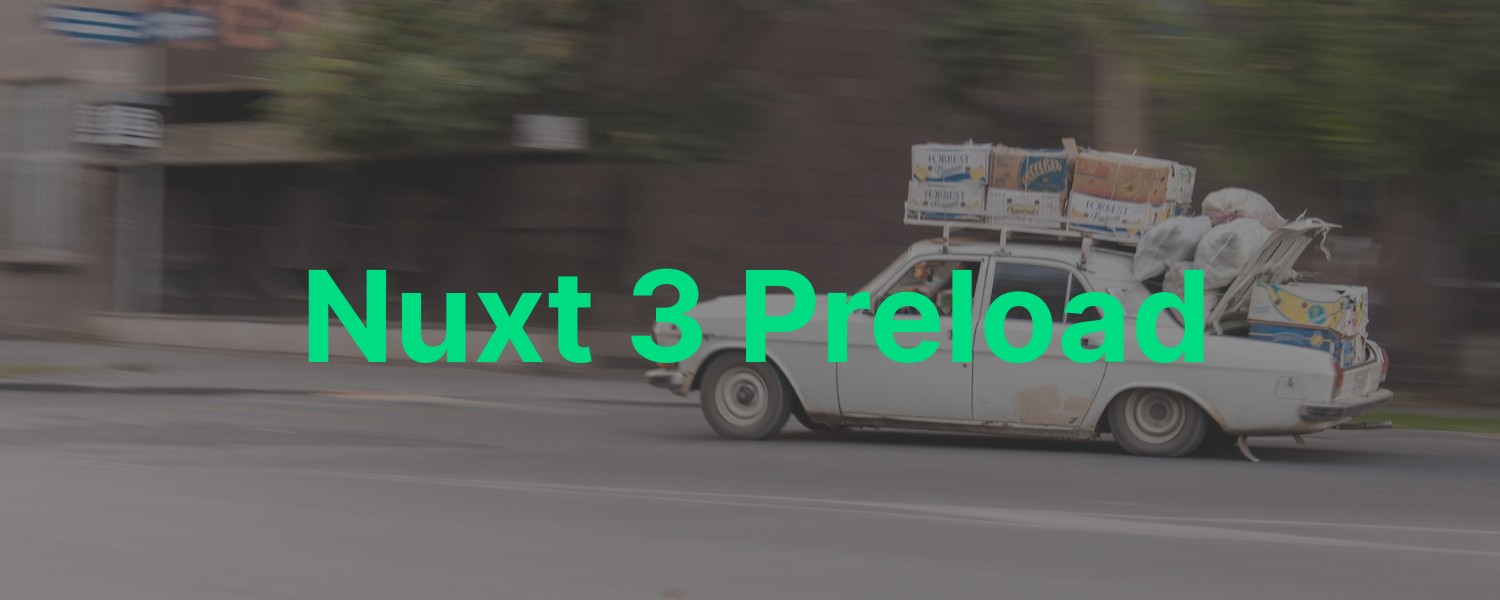
Preload a route or component programmatically in Nuxt 3
Discover how to preload a specific route when the user is highly likely to navigate to that page, even when the route link isn't currently visible in Nuxt 3.
As you know Nuxt 3
prefetch all Nuxt Links by default, so if you are using <NuxtLink> component, you don't need to do any extra thing to handle prefetching.
But, what if we want to prefetch a specific link based on user behaviors and interactions?
In that case, we need to prefetch routes programmatically.
Scenario
1) Based on the logic that in your Vue app, process can result in success page or failure page, so preloading can be useful to preload success or failure page.
2) Another scenario is that you want to bring a seamless user experience to your application and analytics show that users often move from one page to another in a predictable pattern and you can preload routes based on analytics.
How to Preload
In Nuxt 3, we have access to preloadComponents and preloadRouteComponents utility functions that help us to preload a component or a page manually.
Example 1
We want to show promotion page to user, based on user state. and if user is hover over the element, we want to preload the promotion page.
<script setup lang="ts">
const showPromotionPage = ref(true)
function onHover() {
if (showPromotionPage.value) {
preloadRouteComponents('/promotion-page')
}
}
async function onCLick() {
if (showPromotionPage.value) {
await navigateTo('/promotion-page')
}
else {
await navigateTo('/default-page')
}
}
</script>
<template>
<div>
<button @click="onCLick" @mouseover="onHover">
Button
</button>
</div>
</template>
Now if we check the browser's network tab, we will see promotion page is preloaded after hovering over the button.
Example 2
Preload dashboard page when user wants to login.
<script setup lang="ts">
await preloadRouteComponents('/MyGlobalErrorComponent')
async function submit() {
preloadRouteComponents('/dashboard')
const success = await getProcessResultAPI()
if (success) {
await navigateTo('/dashboard')
}
else {
// showing MyGlobalErrorComponent
showError()
}
}
</script>
<template>
<div>
<button @click="submit">
Start Process
</button>
</div>
</template>
Also, you can combine this with useAsyncData composable to cache the next page data and use the same key to retrieve cached data.
Questions around preloading
Route prefetching is a performance optimization technique that loads route components in advance, before the user actually navigates to that route, reducing perceived loading time.
<NuxtLink>
automatically prefetches route components for links visible in the viewport, improving navigation speed for visible links.
Use programmatic prefetching when you can predict user navigation patterns that aren't visible in the current viewport, like anticipating next steps in a multi-step process.
In Nuxt 3, use preloadRouteComponents()
to manually prefetch route components before navigation.